728x90
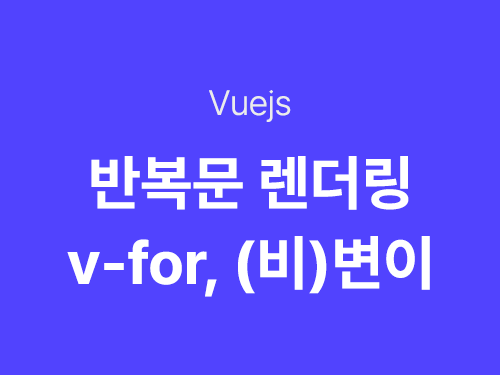
🌲V-for
🌳변수 추가
반복처리할 때 새로운 변수(두 번째 인자)를 추가하는 방법
<template>
<ul>
<li
v-for="(Fruit, index) in Fruits"
:key="Fruit">
{{ Fruit }} - {{ index }}
</li>
</ul>
</template>
<script>
export default {
data () {
return {
Fruits: [ 'Apple', 'Banana', 'Cherry' ]
}
}
}
</script>
Apple - 0
Banana - 1
cherry - 2
🌳고유값 생성
무작위의 고유값을 출력하는 패키지
$ npm i -D shortid
import shortid from 'shortid'
🌵코드 비교 A-B
<template>
<ul>
<li
v-for="Fruit in newFruits"
:key="Fruit.id">
{{ Fruit.name }} - {{ Fruit.id }}
</li>
</ul>
</template>
<script>
export default {
data () {
return {
Fruits: [ 'Apple', 'Banana', 'Cherry' ]
}
},
computed: {
newFruits() {
return this.fruits.map((fruit, index) => {
return {
id: index,
name: fruit
}
}
}
}
}
</script>
<template>
<ul>
<li
v-for="{ id, name } in newFruits"
:key="id">
{{ name }} - {{ id }}
</li>
</ul>
</template>
<script>
import shortid from 'shortid'
export default {
data () {
return {
Fruits: [ 'Apple', 'Banana', 'Cherry' ]
}
},
computed: {
newFruits() {
return this.fruits.map(fruit => ({
id: shortid.generate(),
name: fruit
})
}
}
}
</script>
객체데이터를 갖는다면 객체구조분해 문법을 사용하여 바로 속성값을 사용할 수 있다.
🌲Array Change Detection
배열 변경 감지
🌳변이 메소드
Mutation Methods
배열 변경을 감지하여 바로 적용되는 반응성을 갖는다.
push, pop, shift, unshift, splice, sort, reverse등의 메소드가 있다.
(예시) Fruits라는 배열에 마지막 데이터로 Orange를 추가
methods: {
handler(){
this.Fruits.push('Orange')
{
}
이처럼 원본의 배열에 변형이 일어난다
🌳비변이 메소드
변이 메소드는 호출된 원래 배열을 변경하는 반면 filter, concat, slice는 원본은 변형하지 않고 새로운 배열로 바꿔 화면을 갱신한다.
728x90
'FE' 카테고리의 다른 글
Vue.js 이벤트 핸들링, 이벤트 수식어, 키 수식어 (0) | 2021.12.20 |
---|---|
Vue.js 인스턴스와 라이프사이클 훅 (0) | 2021.12.20 |
Vue.js 조건부 렌더링 v-if, v-show (0) | 2021.12.11 |
Vue.js class, style binding (0) | 2021.12.11 |
Vue.js Watch/Computed Caching - Computed VS Methods (0) | 2021.12.11 |